- Home
- /
- Digitalization
- /
- Mastering SOLID Principles
Introduction
The world of software development thrives on principles that ensure robust, scalable, and maintainable code. Among these, the SOLID principles stand out as a cornerstone of object-oriented programming (OOP). Crafted by Robert C. Martin (Uncle Bob), these five principles guide developers in writing clean and efficient code, simplifying complex systems while promoting adaptability and resilience.
This article unpacks the SOLID principles with real-world examples, case studies, and answers to frequently asked questions to empower you to apply these principles in your projects.
What Are the SOLID Principles?
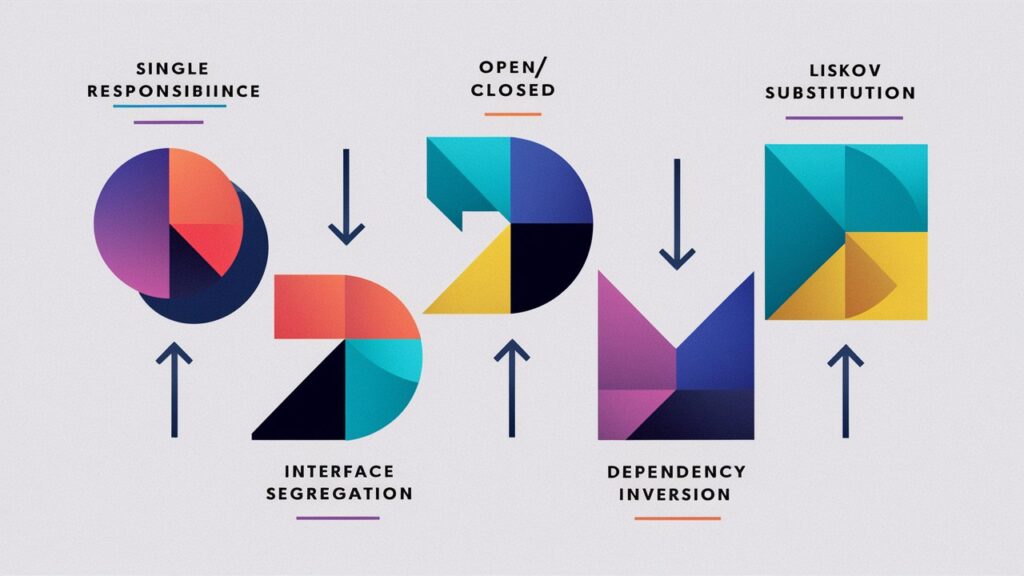
SOLID is an acronym representing five foundational principles for OOP:
- Single Responsibility Principle (SRP)
- Open-Closed Principle (OCP)
- Liskov Substitution Principle (LSP)
- Interface Segregation Principle (ISP)
- Dependency Inversion Principle (DIP)
Why SOLID Principles Matter
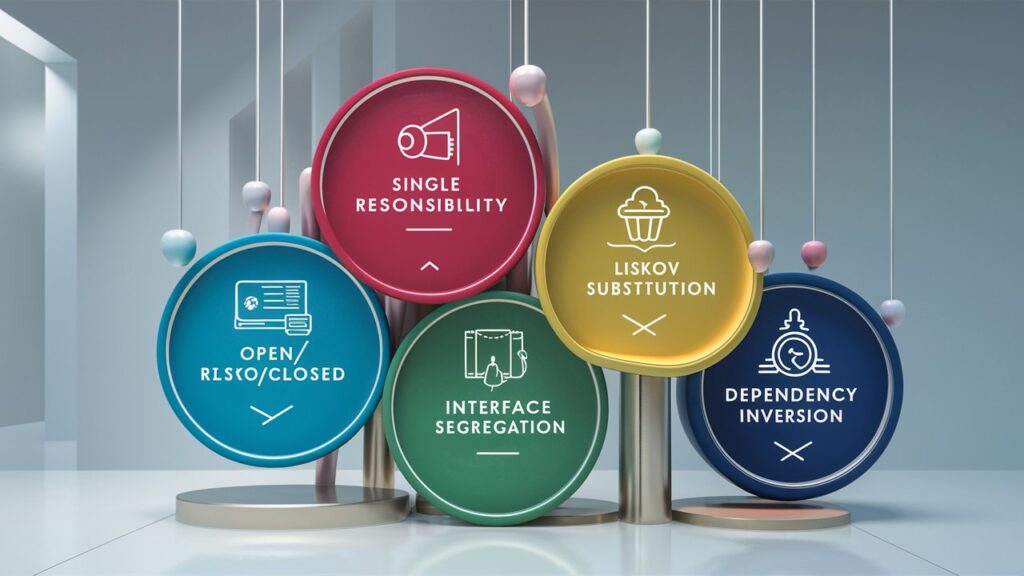
Applying SOLID principles results in:
- Cleaner code that is easier to understand and maintain.
- Reduced development costs due to fewer bugs and quicker feature additions.
- Scalability that supports growing requirements without drastic rewrites.
About Each Principle
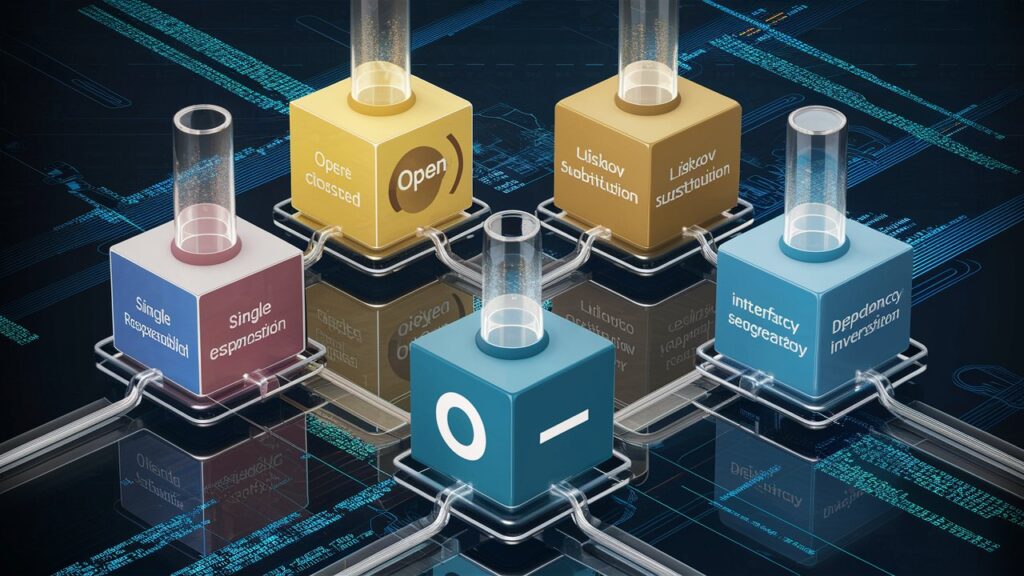
1. Single Responsibility Principle (SRP)
A class should have only one reason to change.
Each class in your codebase should focus on a single task or responsibility. This makes the system easier to debug and reduces the risk of unintended consequences when modifying the class.
Example
Imagine an Invoice
class that calculates totals, formats invoices, and sends emails. This violates SRP. Instead, split the responsibilities:
InvoiceCalculator
handles calculations.InvoiceFormatter
formats the invoice.EmailSender
sends the invoice.
2. Open-Closed Principle (OCP)
A class should be open for extension but closed for modification.
Enhancements to a class’s behavior should not require altering its source code, as this could introduce bugs. Instead, use inheritance or interfaces.
Example
A notification system sends alerts via email. Extending it to support SMS should not involve modifying the email notification code. Use polymorphism:
pythonCopy codeclass Notification:
def send(self, message): pass
class EmailNotification(Notification):
def send(self, message): print("Email:", message)
class SMSNotification(Notification):
def send(self, message): print("SMS:", message)
3. Liskov Substitution Principle (LSP)
Subtypes must be substitutable for their base types.
Derived classes must retain the functionality of their base classes without altering expected behaviors.
Case Study
A media player app has a MediaPlayer
base class with a play()
method. A subclass, VideoPlayer
, overrides play()
but also introduces a loadVideo()
method, breaking LSP. If a VideoPlayer
is used where a MediaPlayer
is expected, the system may crash.
Solution
Ensure the VideoPlayer
strictly adheres to MediaPlayer
’s expected behavior.
4. Interface Segregation Principle (ISP)
A class should not be forced to implement interfaces it does not use.
Avoid bloated interfaces that require implementing unnecessary methods. Instead, create smaller, more focused interfaces.
Example
An Animal
interface with methods fly()
, run()
, and swim()
forces all subclasses to implement these methods, even when they don’t apply (e.g., Penguin
).
Solution
Split into specific interfaces:
Flyable
for flying animals.Runnable
for running animals.Swimmable
for swimming animals.
5. Dependency Inversion Principle (DIP)
High-level modules should not depend on low-level modules. Both should depend on abstractions.
DIP reduces coupling by making modules depend on abstractions rather than concrete implementations.
Example
A payment processing system directly references a CreditCardProcessor
class. Adding PayPalProcessor
requires modifying existing code.
Solution
Introduce an abstraction:
pythonCopy codeclass PaymentProcessor:
def process_payment(self): pass
class CreditCardProcessor(PaymentProcessor):
def process_payment(self): print("Processing credit card")
class PayPalProcessor(PaymentProcessor):
def process_payment(self): print("Processing PayPal")
Real-World Case Studies
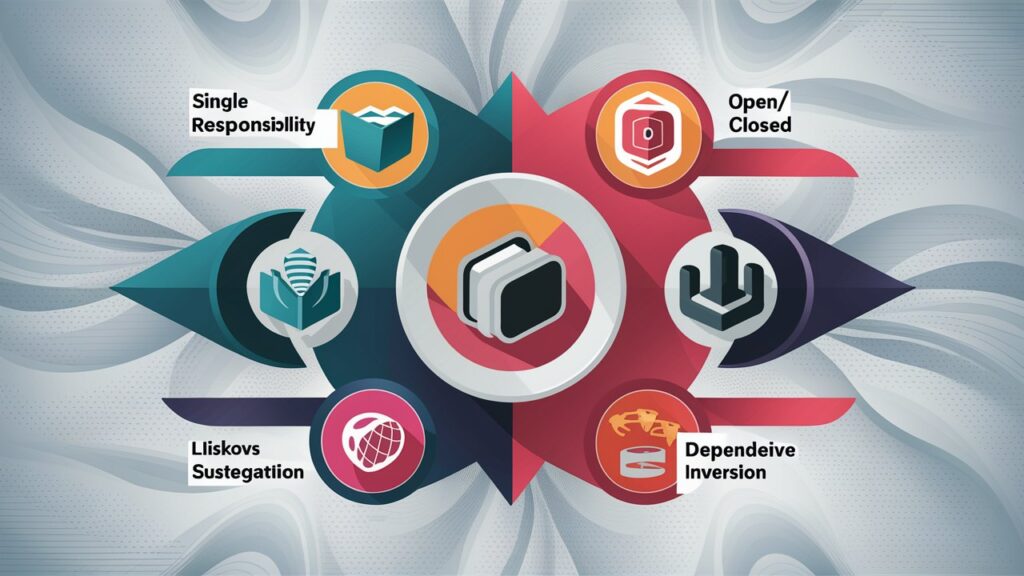
Case Study 1: E-Commerce Platform
An e-commerce giant struggled with adding payment gateways. Adopting DIP, they introduced a PaymentGateway
interface, enabling seamless integration of new gateways without disrupting existing functionality.
Case Study 2: Ride-Hailing App
A ride-hailing app faced difficulty updating vehicle types. Following OCP, they designed a Vehicle
base class with specific subclasses (Car
, Bike
). Adding new vehicle types like ElectricScooter
required only new classes, leaving the existing code untouched.
Frequently Asked Questions
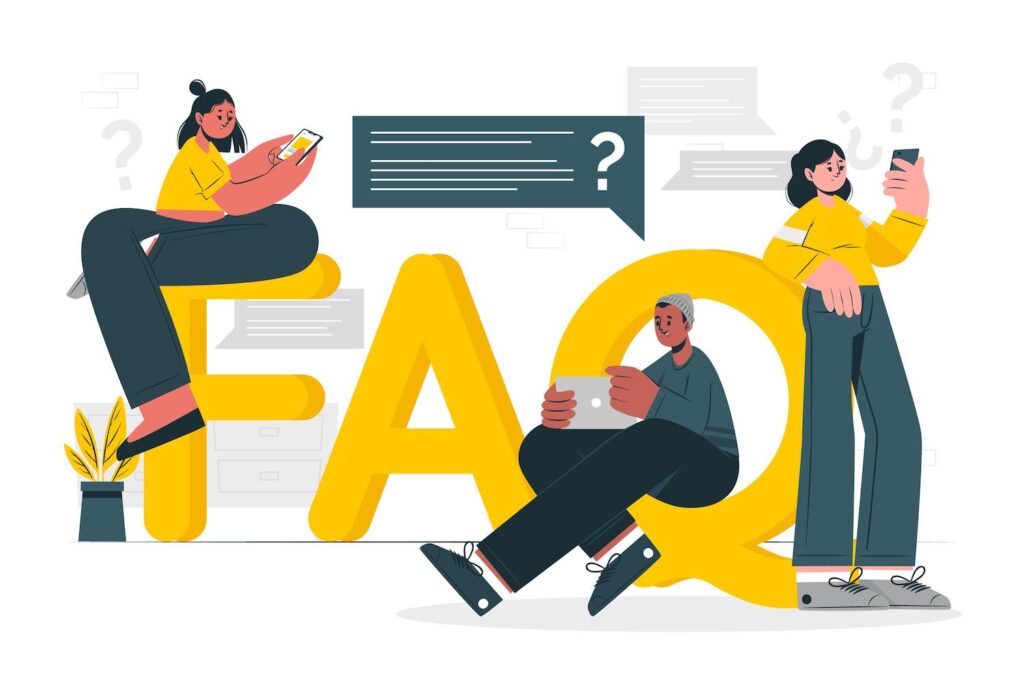
1. Are SOLID principles applicable to non-OOP languages?
While SOLID is tailored for OOP, its core ideas—like separation of concerns and modular design—can be applied in other paradigms.
2. Can following SOLID principles lead to overengineering?
Yes, if misapplied. Use them judiciously, focusing on solving current issues rather than preempting hypothetical ones.
3. Are SOLID principles relevant in agile development?
Absolutely. Agile thrives on adaptability, and SOLID principles ensure code remains flexible and robust against frequent changes.
Key Takeaways
- SOLID principles empower developers to build scalable, maintainable systems.
- Overcoming initial complexity pays off in reduced technical debt and enhanced agility.
- Start small: Apply SRP and OCP to achieve immediate benefits and scale to other principles over time.
Recommended Books and Resources

- Clean Code by Robert C. Martin
- Design Patterns: Elements of Reusable Object-Oriented Software by the Gang of Four
- Clean Architecture by Robert C. Martin
By mastering SOLID principles, you can transform how you design, write, and maintain software, ensuring your projects remain a joy to work on for years to come.
Conclusion
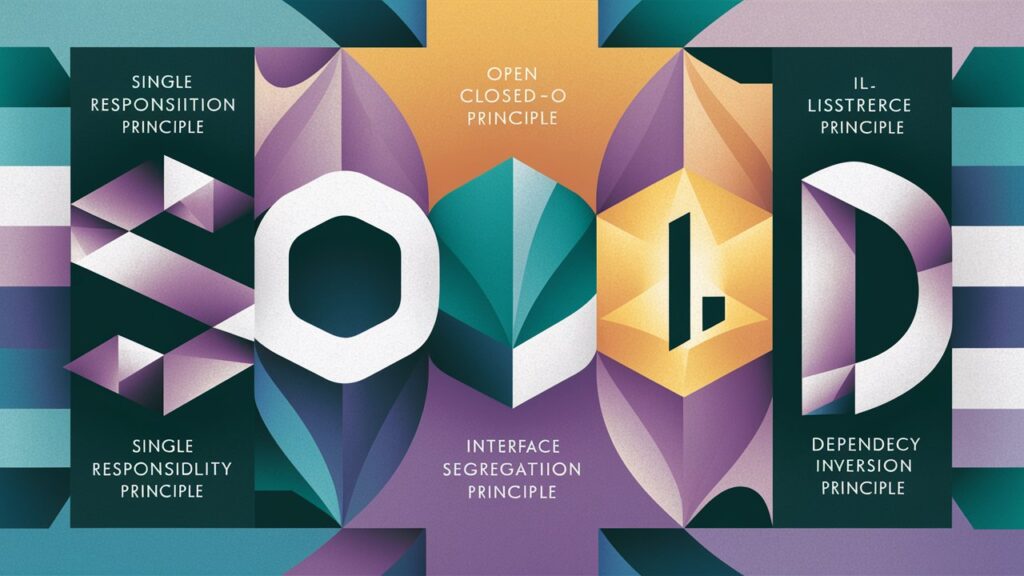
The SOLID principles serve as a guiding light for software developers striving to build systems that are clean, scalable, and easy to maintain. By adhering to these principles, you can reduce technical debt, improve collaboration among teams, and adapt to changing requirements with ease. However, as with any methodology, it’s essential to apply SOLID thoughtfully, balancing the principles with practical needs and project constraints.
Embracing SOLID is not just about writing better code—it’s about fostering a mindset that prioritizes clarity, flexibility, and long-term success. Start small, integrate these principles into your workflow, and watch as they transform your development process into one that stands the test of time.
Enjoyed this article? Dive into Microservices, learn about Cloud Computing, or satisfy your curiosity about DevOps.
Leave a Reply